Python 列表(List)
序列是Python中最基本的数据结构。序列中的每个元素都分配一个数字- 它的位置,或索引,第一个索引是0,第二个索引是1,依此类推。
Python有6个序列的内置类型,但最常见的是列表和元组。
序列都可以进行的操作包括索引,切片,加,乘,检查成员。
此外,Python已经内置确定序列的长度以及确定最大和最小的元素的方法。
列表是最常用的Python数据类型,它可以作为一个方括号内的逗号分隔值出现。
列表的数据项不需要具有相同的类型
创建一个列表,只要把逗号分隔的不同的数据项使用方括号括起来即可。如下所示:
list1 = ['physics', 'chemistry', 1997, 2000];
list2 = [1, 2, 3, 4, 5 ];
list3 = ["a", "b", "c", "d"];
与字符串的索引一样,列表索引从0开始。列表可以进行截取、组合等。
将一个字符串变成列表的方法:
方法一:>>>a
'abcdefghijklmnopqrstuvwxyz'
>>>list(a)
['a', 'b','c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r','s', 't', 'u', 'v', 'w', 'x', 'y', 'z']
方法二:
>>>a
'abcdefghijklmnopqrstuvwxyz'
>>>b=[]
>>>b.extend(a)
>>>b
['a', 'b','c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r','s', 't', 'u', 'v', 'w', 'x', 'y', 'z']
将列表转变成字符串:
>>> a
['a', 'b','c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r','s', 't', 'u', 'v', 'w', 'x', 'y', 'z']
>>>''.join(a)
'abcdefghijklmnopqrstuvwxyz'
>>>'.'.join(a)
'a.b.c.d.e.f.g.h.i.j.k.l.m.n.o.p.q.r.s.t.u.v.w.x.y.z'
>>>'_'.join(a)
'a_b_c_d_e_f_g_h_i_j_k_l_m_n_o_p_q_r_s_t_u_v_w_x_y_z'
访问列表中的值
使用下标索引来访问列表中的值,同样你也可以使用方括号的形式截取字符,如下所示:
#!/usr/bin/python
list1 = ['physics', 'chemistry', 1997, 2000];
list2 = [1, 2, 3, 4, 5, 6, 7 ];
print "list1[0]: ", list1[0]
print "list2[1:5]: ", list2[1:5]
以上实例输出结果:
list1[0]: physics
list2[1:5]: [2, 3, 4, 5]
更新列表
你可以对列表的数据项进行修改或更新,你也可以使用append()方法来添加列表项,如下所示:
#!/usr/bin/python
list = ['physics', 'chemistry', 1997, 2000];
print "Value available at index 2 : "
print list[2];
list[2] = 2001;
print "New value available at index 2 : "
print list[2];
注意:我们会在接下来的章节讨论append()方法的使用
以上实例输出结果:
Value available at index 2 :
1997
New value available at index 2 :
2001
删除列表元素
可以使用 del 语句来删除列表的的元素,如下实例:
#!/usr/bin/python
list1 = ['physics', 'chemistry', 1997, 2000];
print list1;
del list1[2];
print "After deleting value at index 2 : "
print list1;
以上实例输出结果:
['physics', 'chemistry', 1997, 2000]
After deleting value at index 2 :
['physics', 'chemistry', 2000]
删除指定范围的元素:
>>> a=range(40)
>>> a
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13,14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33,34, 35, 36, 37, 38, 39]
>>> a[6:11]
[6, 7, 8, 9, 10]
>>> del a[6:11]
>>> a
[0, 1, 2, 3, 4, 5, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24,25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39]
注意:我们会在接下来的章节讨论remove()方法的使用
Python列表脚本操作符
列表对 + 和 * 的操作符与字符串相似。+ 号用于组合列表,*号用于重复列表。
如下所示:
Python 表达式 | 结果 | 描述 |
len([1, 2, 3]) | 3 | 长度 |
[1, 2, 3] + [4, 5, 6] | [1, 2, 3, 4, 5, 6] | 组合 |
['Hi!'] * 4 | ['Hi!', 'Hi!', 'Hi!', 'Hi!'] | 重复 |
3 in [1, 2, 3] | True | 元素是否存在于列表中 |
for x in [1, 2, 3]: print x, | 1 2 3 | 迭代 |
Python列表截取
Python的列表截取与字符串操作类型,如下所示:
L = ['spam', 'Spam', 'SPAM!']
操作:
Python 表达式 | 结果 | 描述 |
L[2] | 'SPAM!' | 读取列表中第三个元素 |
L[-2] | 'Spam' | 读取列表中倒数第二个元素 |
L[1:] | ['Spam', 'SPAM!'] | 从第二个元素开始截取列表 |
|
|
|
最列表最后5个:
>>> names[-5:]
[995, 996, 997, 998, 999]
Python列表函数&方法
Python包含以下函数:
序号 | 函数 |
1 | cmp(list1, list2) |
2 | len(list) |
3 | max(list) |
4 | min(list) |
5 | list(seq) |
Python包含以下方法:
序号 | 方法 |
1 | list.append(obj) |
2 | list.count(obj) |
3 | list.extend(seq) |
4 | list.index(obj) |
5 | list.insert(index, obj) |
6 | list.pop(obj=list[-1]) |
7 | list.remove(obj) |
8 | list.reverse() |
9 | list.sort([func]) |
例子1:
names=['IBM','Alex','JACK','DELL']
#names=[]
names.extend(range(1000))
#names = names+range(1000)
names.insert(100,'IBM')
names.insert(300,'IBM')
names.insert(500,'IBM')
for i in range(names.count('IBM')):
#print i
ibm_index=names.index('IBM')
print 'INDEX:',ibm_index
names[ibm_index]='HP'
print names.count('HP')
例子2
#!/usr/bin/env python
import sys
salary=int(raw_input('Please input your salary:'))
products=[['Iphone',5800],['MacPro',12000],['NB Shoes',680],['Cigrate',48],['MX4',2500]]
#create a shopping list
shopping_list=[]
while True:
for p in products:
print products.index(p), p[0],p[1]
choice = raw_input("\033[32;1mPlease choose sth to buy:\033[0m").strip()
if choice == 'quit':
print "You have bought below stuff:"
for i in shopping_list:
print '\t',i
sys.exit('Goodbye!')
if len(choice) == 0:continue
if not choice.isdigit():continue
choice = int(choice)
if choice >= len(products): #out of range
print '\033[31;1mCould not find this item\033[0m'
continue
pro = products[choice]
if salary >= pro[1]: #means you can afford this
salary=salary - pro[1]
shopping_list.append(pro)
print "\033[34;1mAdding %s to shopping list,you have %s left\033[0m" %(pro[0],salary)
else:
print 'The price of %s is %s,yet your current balance is %s,so try another one!'%(pro[0],pro[1],salary)
列子3:
模糊查询姓名并高亮
#!/usr/bin/env python
staff_dic={}
f=file('stu_info.txt')
for line in f.xreadlines():
stu_id,stu_name,mail,company,title,phone=line.split()
staff_dic[stu_id]=[stu_name,mail,company,title,phone]
while True:
query=raw_input('\033[32;1mPlease input the querystring\033[0m').strip()
if len(query)< 3:
print 'you haveto input at least 3 letters to query'
continue
match_counter = 0
for k,v instaff_dic.items():
index=k.find(query)
if index != -1:
printk[:index]+ '\033[32;1m%s\033[0m' %query +k[index+len(query):],v
match_counter+=1
else:
str_v='\t'.join(v)
index=str_v.find(query)
if index != -1:
printk,str_v[:index]+ '\033[32;1m%s\033[0m' %query +str_v[index+len(query):]
match_counter +=1
# for i inv:#going to do the fuzzy matchs
# ifi.find(query) !=-1:#find item
# str_v='\t'.join(v)
# printk,str_v
# match_counter +=1
# break
print 'Matched\033[31;1m%s\033[0m records!' %match_counter

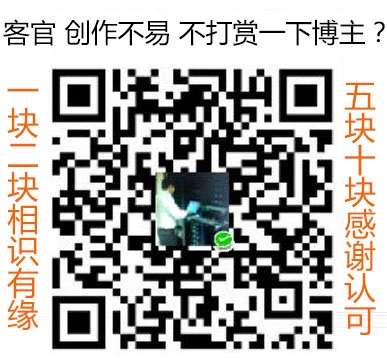
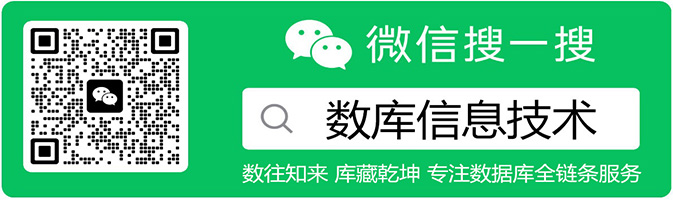